Eloquent Filtering
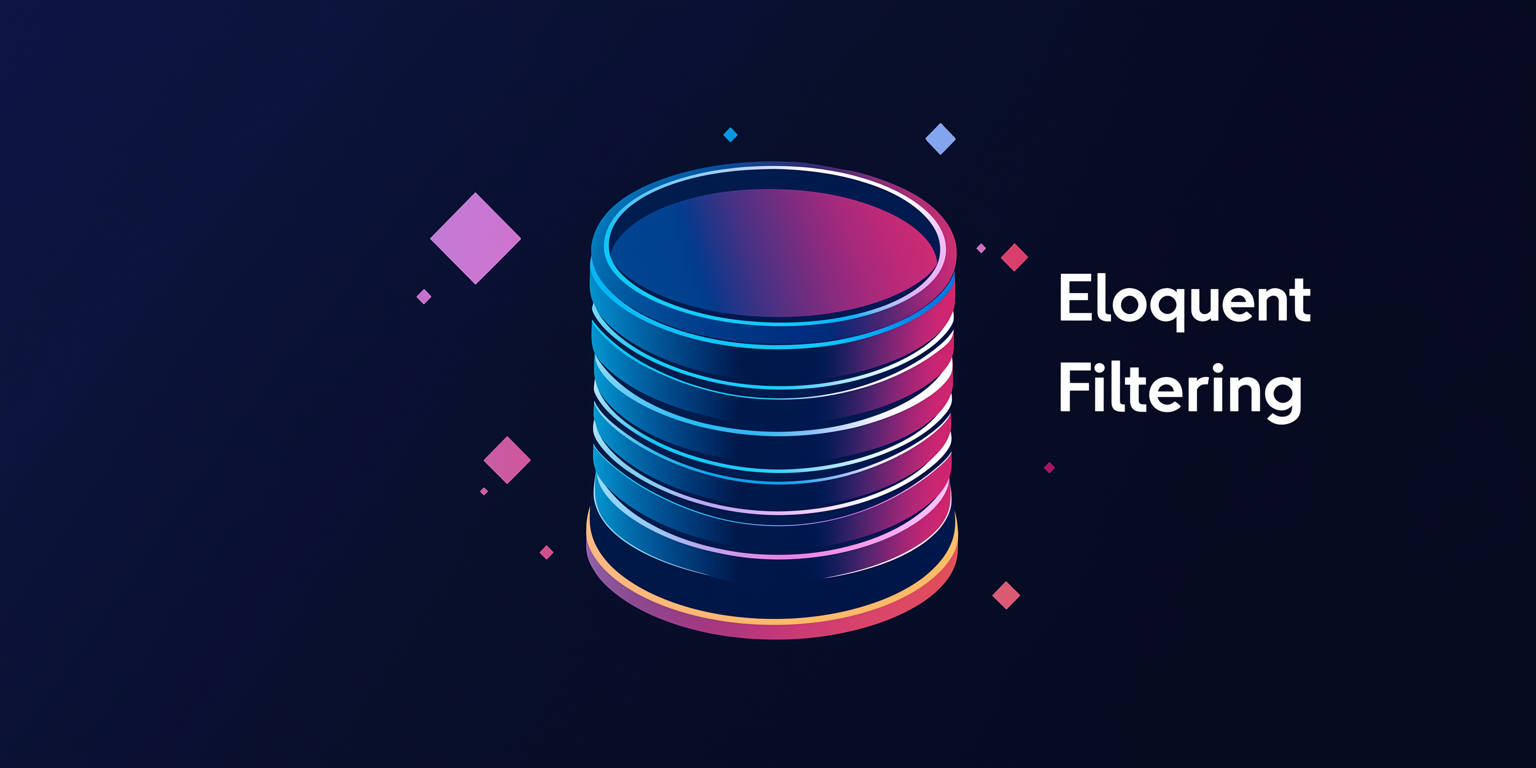
Overview
Eloquent Filtering simplifies implementing search functionality for your Eloquent models, whether simple or complex, by eliminating the need for custom query logic. It allows you to easily define and manage filters directly within your models, and seamlessly apply them using incoming HTTP request data to dynamically filter your models.
With this package, you can build more readable, maintainable, and scalable code, boosting your productivity and speeding up development.
Whether you’re building APIs, dashboards, or advanced search systems, Eloquent Filtering provides a powerful and flexible way to streamline your Eloquent queries, making it easier to manage and extend your application’s filtering capabilities.
Quick Look
Features
Large Filter Library
Many core filter methods.
Field Filters
Filter your models fields.
Relationship Filters
Filter by relationship existence.
Pivot Filters
Filter your intermediate table.
Required Filters
Set filters as required.
Validation
Add validation to your defined filters.
Custom Filters
Create your own custom filters.
Alias
Alias fields and relationships.
Filter JSON Columns
Json path wildcard support.
Granular Control
Specify filter types.